Constant management: practices and strategies
In software development, constants are used to store fixed data that remains the same throughout the program’s execution. The definition of constant is simple. However, managing constants in complicated projects can be a headache sometimes. I worked on the project Agent Portal(AP), and we had a technical debt on constant management. This blog post will talk about strategies we use to manage constants in AP, as well as some other strategies that people use to manage constants in software applications.
Constant management in AP
A good constant should have at least the following characteristics
- It stores fixed values that have been used multiple times in different places in the codebase.
- It has a meaningful name. Usually the name describes the purpose of constant or the data they represent.
- It has a scope.
- It has a specific data type.
As a result, I get a React functional component that uses TypeScript. Here are the type annotations that I added. They clearly indicate the types of each prop.
This is also a guide in AP when we create a constant. In AP, we manage constants from two scopes: global and local. Global constants are usually shared in different components, and local constants are usually for first level or second level components. All constants are started as a local constant, and the name convention for the constant file is <component-name>.constants.ts
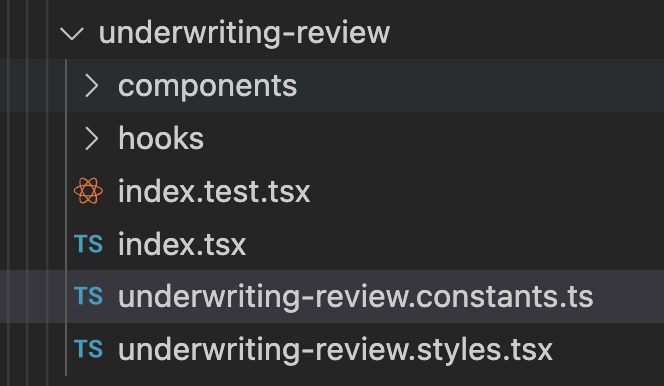
At global level, there is a file called constants.ts
that imports and exports all local constants files, which makes it easier to share local constants across the components. This global constant file also contains project level constants which clearly the scope is global.
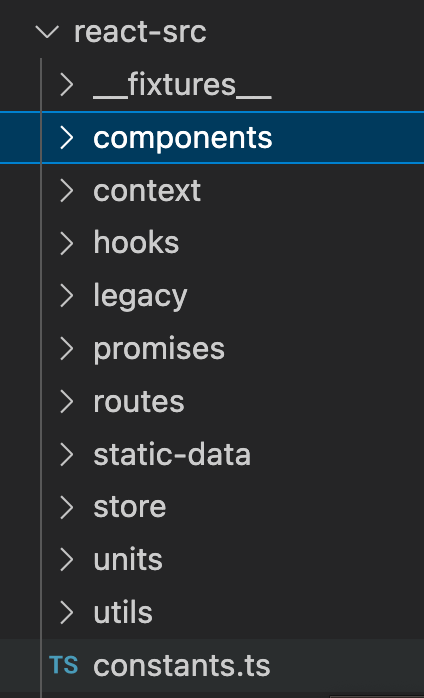
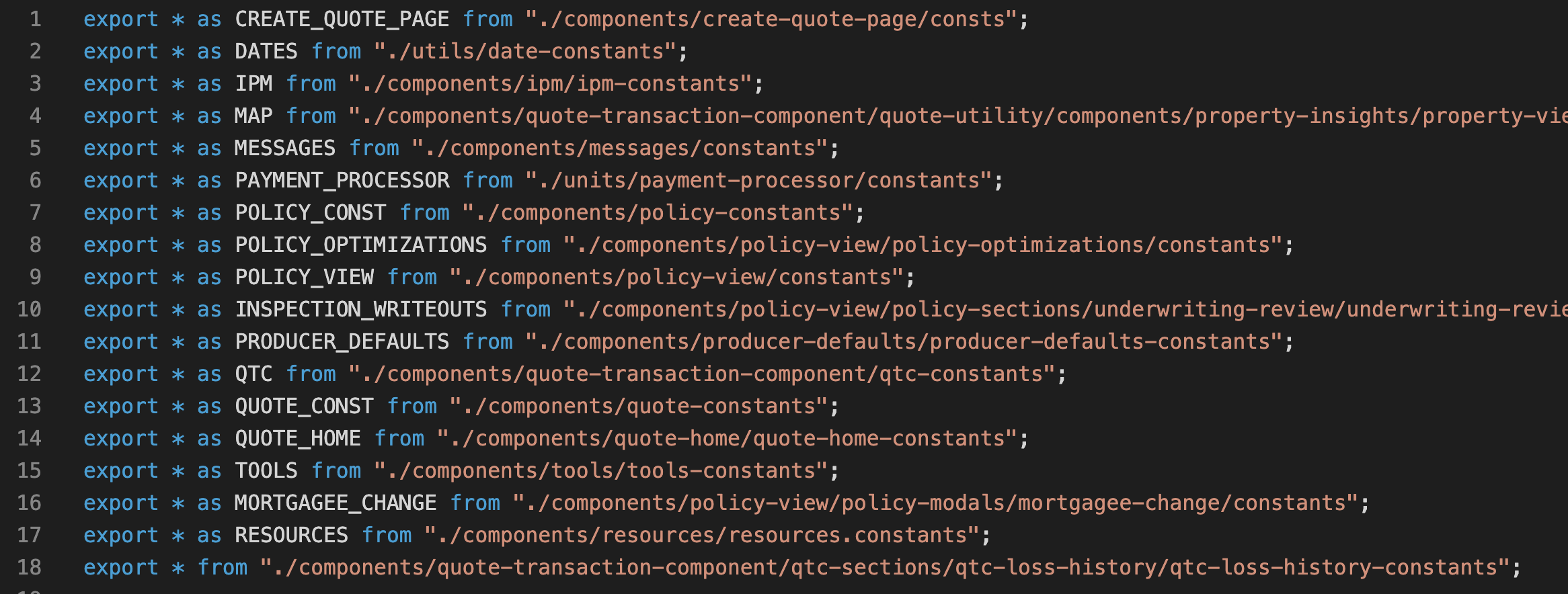
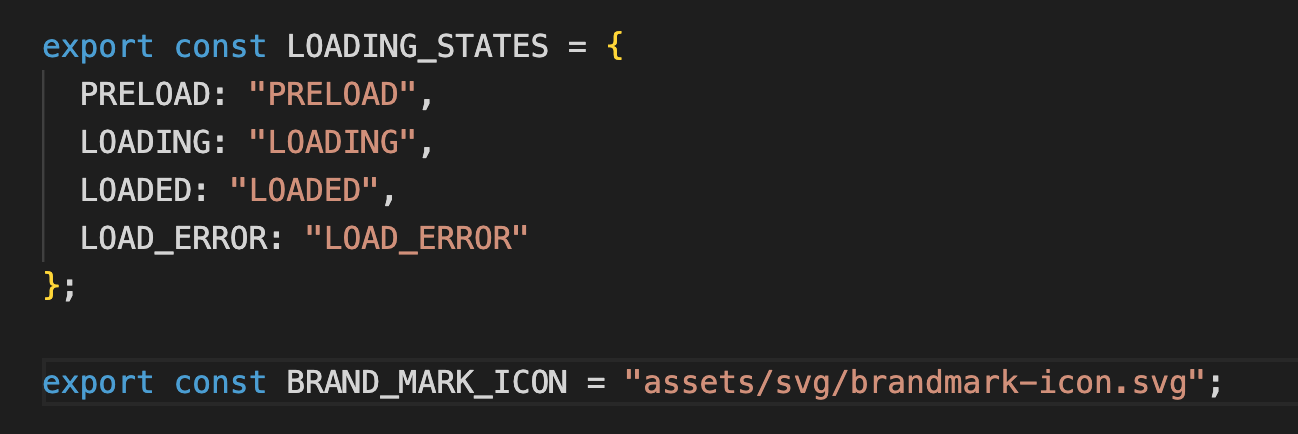
The pros of this strategy is that it’s easy to locate constants and all constants are rooted from one place. This approach also simplifies updates and modifications.
The cons is that it requires a clear and thoughtful categorization scheme. Be Careful when introducing the new constants, and give it the right scope.
Other strategies to manage constants
1. Centralized Constants File:
Pros:
- Easy to locate and manage constants in a single file.
- Enhances consistency as all constants are in one place.
- Simplifies updates and modifications.
Cons:
- Can become overwhelming as the codebase grows.
- May require careful organization and categorization to maintain readability.
2. External Configuration:
Pros:
- Allows for configuration adjustments without modifying the code.
- Useful for constants that change based on different environments (e.g., URLs, API keys).
- Separates concerns by keeping configuration separate from application logic.
Cons:
- Introduces external dependencies and potential configuration errors.
- Might lead to confusion if not well-documented or version-controlled.
- Using Frameworks or Libraries:
Pros:
- Leverage established practices and patterns for managing constants.
- Frameworks may provide additional tools for validation, configuration, etc.
Cons:
- May introduce a learning curve for using the specific framework.
- Could lead to unnecessary dependencies if not carefully considered.
- Application-Specific Constants Classes:
Pros:
- Encapsulates constants within classes, offering better organization.
- Provides a clear naming convention by using class namespaces.
Cons:
- Might introduce additional complexity for small projects with limited constants.
- Requires adherence to the class-based approach throughout the codebase.
- Enums (Enumerations):
Pros:
- Provides a clear and expressive way to represent related constants.
- Enhances type safety as enums restrict values to a predefined set.
- Reduces the chance of using arbitrary integer or string values.
Cons:
- Limited flexibility in cases where the values are not enumerable.
- Adds some complexity when compared to simple numeric or string constants.
- Database or External Storage:
Pros:
- Offers the flexibility to change constants without redeploying the application.
- Useful for frequently changing values or for non-technical users to manage.
Cons:
- Introduces performance overhead when fetching values from an external source.
- Can complicate deployment and version control, especially in distributed systems.
Conclusion
In conclusion, there is no one-size-fits-all strategy for managing constants. The best approach depends on your project’s size, complexity, team dynamics, and future scalability requirements. A combination of these strategies might be necessary to strike the right balance between maintainability, readability, and flexibility. Whichever strategy you choose, ensure that your team is aligned and that the chosen approach is well-documented to facilitate seamless collaboration and future development.